728x90
백준 2231번 분해합
- 레벨 : 골드 2
- class : 2
📖 문제
생성자 245
분해합 245 + 2 + 4 + 5 = 256
자연수 N은 1에서 1,000,000 사이의 수만 입력된다.
N의 생성자를 출력하시오.
만약, 생성자가 없는 경우, 0을 출력한다.
📖 예제 입력 1
216
📖 예제 출력 1
198
📝제출 코드1
- 언어 Java11
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class Main {
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
String str = br.readLine();
int n = Integer.parseInt(str);
br.close();
int nCopy = n;
int min = 1000001;
while(nCopy>0) {
String ncStr = Integer.toString(nCopy);
String[] array = ncStr.split("");
int sum =0;
for(int i=0; i<array.length; i++) {
sum += Integer.parseInt(array[i]);
}
if(n == nCopy + sum) {
min = Math.min(min, nCopy);
}
nCopy--;
}
if(min == 1000001)
System.out.println(0);
else
System.out.println(min);
}
}
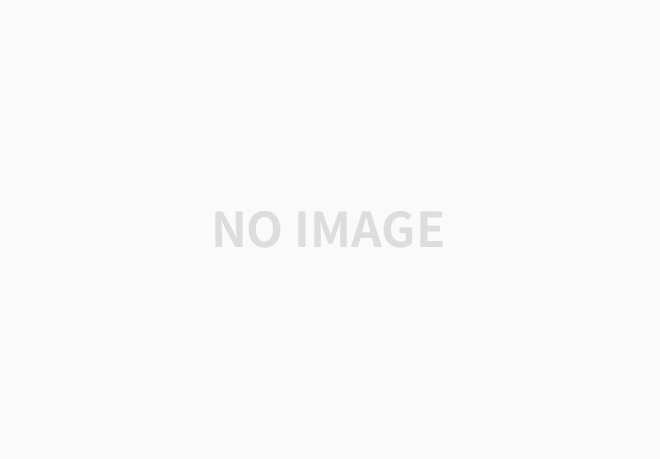
최소값 min 설정을 N입력값이 될 수 없는 1,000,001로 설정해 생성자가 없는 경우를 분류 했다.
🔎정수를 문자열로 변환
Integer.toString(정수형 변수);
📝제출 코드2
- 언어 Java11
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class Main {
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
String str = br.readLine();
int n = Integer.parseInt(str);
br.close();
int nCopy = n;
int min = 1000001;
while(nCopy>0) {
String ncStr = Integer.toString(nCopy);
int sum =0;
for(int i=0; i<ncStr.length(); i++) {
sum += (ncStr.charAt(i) - '0');
}
if(n == nCopy + sum) {
min = Math.min(min, nCopy);
}
nCopy--;
}
if(min == 1000001)
System.out.println(0);
else
System.out.println(min);
}
}
아까와 같은 로직이지만, 배열을 생성해주지 않고 실행될 수 있게끔 코드를 변환해주었다.
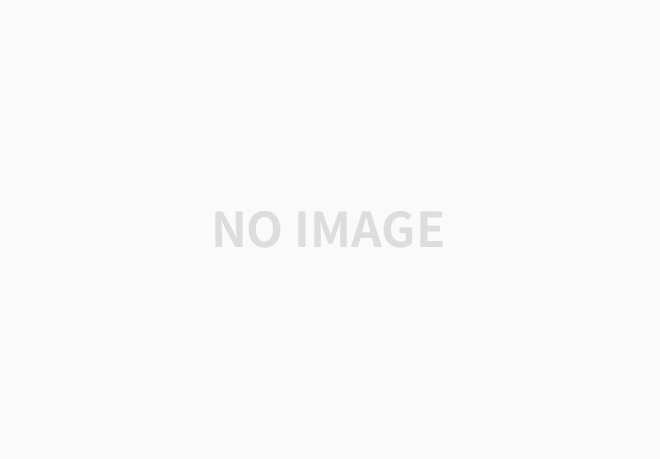
🔎 숫자문자(Character) 를 숫자로 변환
'5' - '0' = 5
str.charAt(i) - '0'
📝공부해볼 코드
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int N = sc.nextInt();
int res = 0;
for(int i=0; i<N; i++) {
int num = i;
int sum = 0;// 각 자릿수 합 변수
while(num != 0) {
sum += num % 10;// 각 자릿수 더하기
num /= 10;
}
// i값과 각 자릿수 누적합이 같을 경우
if(sum + i == N) {
res = i;
break;
}
}
System.out.println(res
);
}
}
🍋느낀점
N의 값의 자릿수를 더하는 알고리즘을 짜려고 했으나, 어려워 문자열로 받은 후에 문자를 추출하는 식으로 계산했다.
채점 후, 다른 사람 코드를 볼 수 있는데 너무 잘 짠 코드가 있어 공부해 봐야겠다.
'알고리즘' 카테고리의 다른 글
자료구조 2주차_이진탐색과 재귀함수 (0) | 2022.11.24 |
---|---|
자료구조 2주차_어레이와 링크드리스트 (0) | 2022.11.24 |
자료구조 1주차_시간복잡도 & 공간복잡도 (0) | 2022.11.23 |
자료구조 1주차_알고리즘과 친해지기 (1) | 2022.11.23 |
백준 10828번 스택 (0) | 2022.11.13 |